# ------------------------------- # ENVIRONMENT CONFIGURATION # ------------------------------- # Set default blocksize for ls, df, du # from this: http://hints.macworld.com/comment.php?mode=view&cid=24491 # ------------------------------------------------------------ export BLOCKSIZE=1k # Add color to terminal, from http://osxdaily.com/2012/02/21/add-color-to-the-terminal-in-mac-os-x/ export CLICOLOR=1 export LSCOLORS=ExFxBxDxCxegedabagacad # Sets default terminal editor to Sublime Text export EDITOR=/usr/bin/nano # Set up $PATH https://unix.stackexchange.com/a/415028 typeset -U path_array # Declare an array # Add your paths path_array=( "/home/derrick/bin" $PATH ) # Join the array elements by ":" PATH=$(IFS=":"; echo "${path_array[*]}") # Export the PATH export PATH # Change Prompt autoload -U colors && colors autoload -U add-zsh-hook export PROMPT="%F{cyan}%n%F{white}@%F{green}%m%F{white}:%F{yellow}%~%F{white}$%f " export PS2="╠═> " # iTerm integration test -e "${HOME}/.iterm2_shell_integration.zsh" && source "${HOME}/.iterm2_shell_integration.zsh" || true # ------------------------------- # Aliases # ------------------------------- # ls: ls, but show all alias ls="ls -a --color" # xzz: xz compress with custom params alias xzz="xz -z -e -T 0 -vv" # ------------------------------- # Functions # ------------------------------- # pbcopy via iTerm2 function pbcopy() { #if which pbcopy >/dev/null 2>&1; then # pbcopy "$@" #else # Replace ^[ with the ASCII escape character local start="\e]1337;CopyToClipboard\a" local end="\e]1337;EndCopy\a" printf "${start}$(cat)${end}" #fi } function update_zshrc() { if curl -s -f -o ~/.zshrc https://derrick.blog/wisp/raspberry-pi-zshrc/raw/; then dos2unix "${HOME}/.zshrc" source "${HOME}/.zshrc" echo "Updated .zshrc successfully" else echo "Failed to download .zshrc from GitHub" fi } # ZSH stuff https://dev.to/manan30/what-is-the-best-zshrc-config-you-have-seen-14id #History setup HISTFILE=$HOME/.zsh_history HISTSIZE=100000 SAVEHIST=$HISTSIZE setopt hist_ignore_all_dups # remove older duplicate entries from history setopt hist_reduce_blanks # remove superfluous blanks from history items setopt inc_append_history # save history entries as soon as they are entered setopt share_history # share history between different instances of the shell setopt correct_all # autocorrect commands setopt auto_list # automatically list choices on ambiguous completion setopt auto_menu # automatically use menu completion setopt always_to_end # move cursor to end if word had one match zstyle ':completion:*' menu select # select completions with arrow keys zstyle ':completion:*' group-name '' # group results by category zstyle ':completion:::::' completer _expand _complete _ignored _approximate #enable approximate matches for completion # ------------------------------- # Prompt Timing Functionality # ------------------------------- # get_current_time_ms: Returns the current time in milliseconds # - Uses $EPOCHREALTIME if available # - Falls back to date with %N option (nanoseconds) if supported # - Falls back to gdate if the above two options are not available function get_current_time_ms() { if [ -n "$EPOCHREALTIME" ]; then echo $EPOCHREALTIME | awk '{printf "%.0f\n", $1*1000}' else time_ns=$(date +%s%N 2>/dev/null) if [[ ${time_ns: -1} != "N" ]]; then echo $(($time_ns/1000000)) else echo $(($(gdate +%s%N)/1000000)) fi fi } # preexec: This function is called before executing any command # - Stores the current time in milliseconds in the 'timer' variable function rprompt_set_start() { rprompt_timer=$(get_current_time_ms) export rprompt_timer; } add-zsh-hook preexec rprompt_set_start # rprompt_timer: This function is called before displaying the prompt # - Calculates the elapsed time of the last command using the 'timer' variable # - Updates the right prompt (RPROMPT) with the elapsed time function rprompt_timer() { if [ "$rprompt_timer" ]; then now=$(get_current_time_ms) elapsed=$((now - rprompt_timer)) reset_color=$'\e[00m' timer_prompt="〔%F{cyan}$(converts "$elapsed")%{$reset_color%}〕" unset rprompt_timer else timer_prompt="" fi # Check if RPROMPT already contains a timer status if [[ "$RPROMPT" == *"〔"* ]]; then # Remove the existing timer status RPROMPT=${RPROMPT#*"〕"} fi # Add the new timer status to the PROMPT RPROMPT="${timer_prompt}${RPROMPT}" export RPROMPT } add-zsh-hook precmd rprompt_timer # converts: This function takes a time value in milliseconds and converts it to # a human-readable format converts() { local t=$1 # Check if the input is a valid number before proceeding with the calculation if ! [[ "$t" =~ ^[0-9]+$ ]]; then echo "converts(): Invalid input $t" return 1 fi # Calculate days, hours, minutes, seconds, and milliseconds from the input time local d=$((t/1000/60/60/24)) local h=$((t/1000/60/60%24)) local m=$((t/1000/60%60)) local s=$((t/1000%60)) local ms=$((t%1000)) # Build and output the human-readable time string if [[ $d -gt 0 ]]; then echo -n "${d}d " fi if [[ $h -gt 0 ]]; then echo -n "${h}h " fi if [[ $m -gt 0 ]]; then echo -n "${m}m " fi if [[ $s -gt 0 ]]; then echo -n "${s}s " fi if [[ $ms -gt 0 ]]; then echo -n "${ms}㎳" fi echo }
.zshrc
Other Posts Not Worth Reading
Hey, You!
Like this kind of garbage? Subscribe for more! I post like once a month or so, unless I found something interesting to write about.
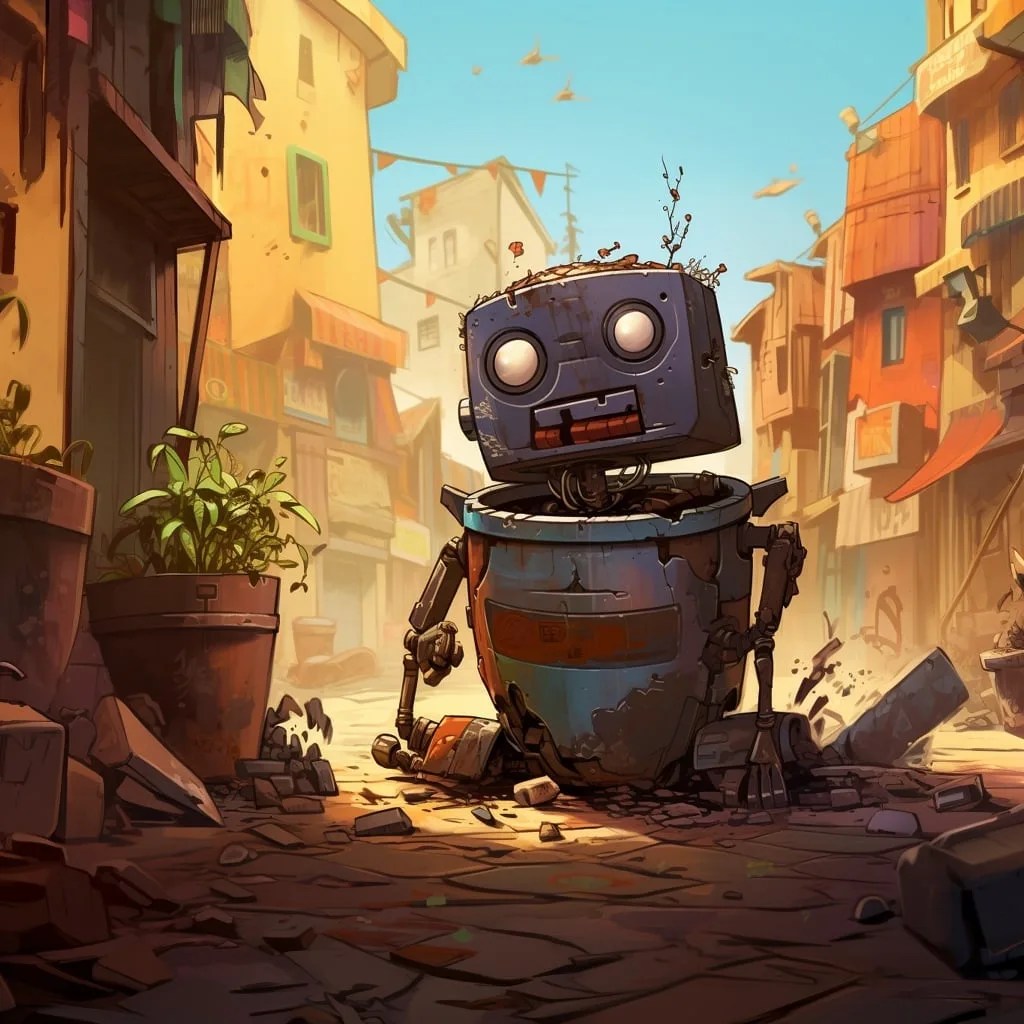
Leave a Reply