As a follow up to my last post about limiting file sizes during uploads, I had to come back to the problem with limiting image sizes for featured images. Not bytes this time, but pixel dimensions.
Still being a bit of a block editor newb, this was an interesting challenge for me, and I was really surprised at how easy it was to implement. I basically googled around and found a few different things to put together that worked for me. The primary source was a post by Igor Benic on how to disable the publish button:
After that, I discovered how to add notifications to the block editor, to tell when the image was too large, thanks to a post by David F. Carr:
So what’s it look like? Well, it’s pretty simple. If you choose a featured image that’s too large for the settings, it will add a non-dismissible error notification to the editor:
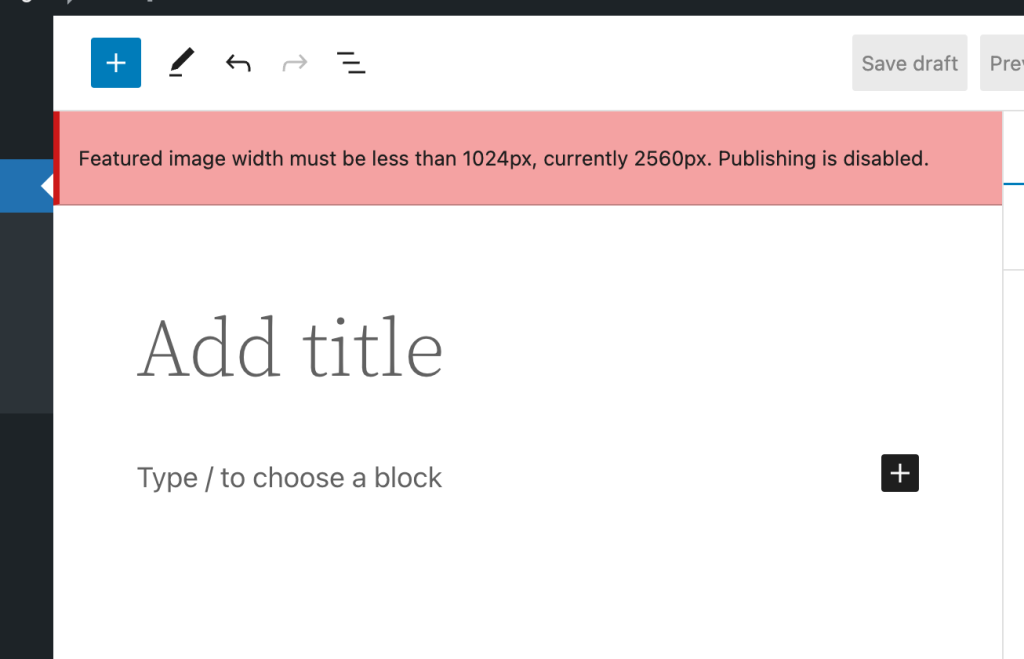
Then it will block you from hitting the publish button:
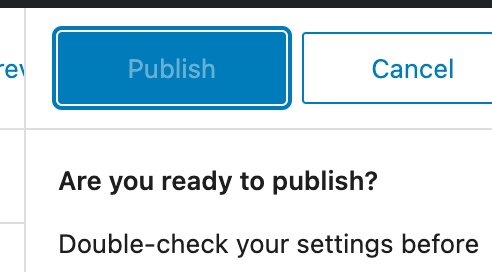
So, finally the code. This is just enqueued in as a simple add_action()
during the enqueue_block_editor_assets
hook. I’m absolutely not a good JS developer, so please don’t judge me too harshly. Also, that means this could be riddled with bugs. Use at your own risk 😀
var postLocked = false;
wp.domReady( () => {
wp.data.subscribe( function() {
var imageId = wp.data.select( 'core/editor' ).getEditedPostAttribute( 'featured_media' ); // Featured Image ID.
// If we have no image ID, and we already locked the post, we won't do anything.
if ( imageId ) {
blockEditorSettings = wp.data.select('core/block-editor').getSettings();
// Default to 1200px wide.
maxImageSize = 1200;
imageAttrs = wp.data.select('core').getMedia( imageId );
// Get the size for the "large image" and if it's available, use that instead.
if ( typeof blockEditorSettings !== 'undefined' && blockEditorSettings.hasOwnProperty( 'imageDimensions' ) ) {
maxImageSize = blockEditorSettings.imageDimensions.large.width;
}
if ( typeof imageAttrs !== 'undefined' && imageAttrs.hasOwnProperty( 'media_details') ) {
// Publish is not locked and width is too large.
if ( ! postLocked && imageAttrs.media_details.width > maxImageSize ) {
postLocked = true;
wp.data.dispatch( 'core/editor' ).lockPostSaving( 'featuredImageTooLarge' );
wp.data.dispatch('core/notices').createNotice(
'error', // Can be one of: success, info, warning, error.
wp.i18n.__( wp.i18n.sprintf( 'Featured image width must be less than %spx, currently %spx. Publishing is disabled.', maxImageSize, imageAttrs.media_details.width ) ),
{
id: 'featuredImageTooLarge', // Assigning an ID prevents the notice from being added repeatedly.
isDismissible: false, // Whether the user can dismiss the notice.
}
);
}
}
} else if ( postLocked ) {
postLocked = false;
wp.data.dispatch( 'core/editor' ).unlockPostSaving( 'featuredImageTooLarge' );
wp.data.dispatch('core/notices').removeNotice( 'featuredImageTooLarge' );
}
} );
} );
Code language: JavaScript (javascript)
Leave a Reply